Passing parameters to imported function in Node.js
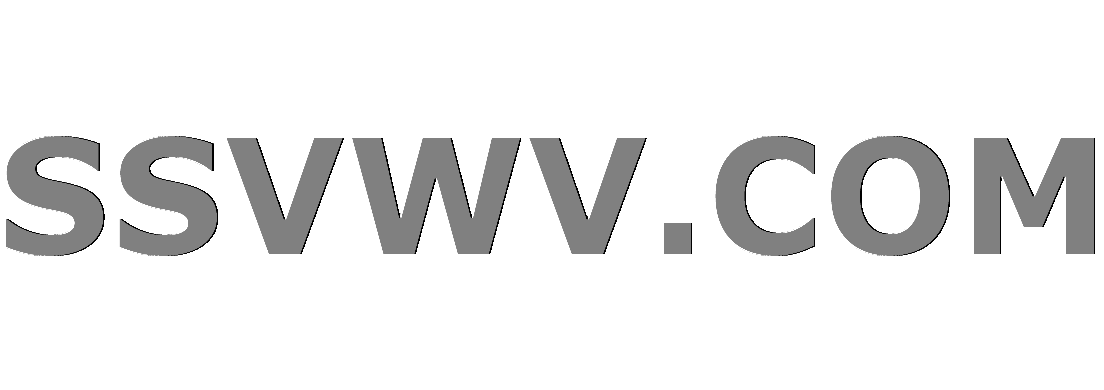
Multi tool use
Passing parameters to imported function in Node.js
I feel like this shouldn't be hard, but I'm new to Node.js (and also new to many aspects of JavaScript). I'm creating an Express application to return arrays of bike locations that are fetched from multiple APIs; each API requires the longitude and latitude as inputs. So I've broken each API call into a 'module' and I'm using the npm library 'async' to make each call in parallel, and using 'axios' to make the API requests. I can get it to work just fine without breaking it up into modules, but once I separate each API call into its own file, I can't figure out how to pass lat and lng into it.
Here is my index.js
import async from 'async';
import {mobike} from './mobike.js';
import {spin} from './spin.js';
async.parallel([
mobike, //How or where can I pass these parameters?
spin
],
function(err, results) {
console.log(results);
});
and here is my mobike.js module, for example (I'll omit spin.js for brevity)
import axios from 'axios';
export var mobike = function (lat, lng, callback){
axios.get('https://mwx.mobike.com/mobike-api/rent/nearbyBikesInfo.do', {
params: {
latitude: lat, //35.2286324
longitude: lng //-80.8427562
},
headers: {
'content-type': 'application/x-www-form-urlencoded',
'user-agent': 'Mozilla/5.0'
}
}).then( response => {
callback(null, response.data.object)
}).catch(error => {
callback(error, null)
})
}
When I try and pass arguments through mobike (like mobike(1234,1234)
), it doesn't work. How can I pass the lat and lng arguments to the mobike.js file?
mobike(1234,1234)
async
promise
Promise.all
Thanks @BrijeshPatel...if I did that, I would no longer require the
async.js
dependency, right?– BYates
Jul 3 at 13:07
async.js
3 Answers
3
From a tutorial I found online, it looks like you need to wrap your mobike
and spin
functions inside other functions in order to bind in your data along with the callback function provided by the async
module. Something like this:
mobike
spin
async
var lat = 35.2286324;
var lng = -80.8427562;
async.parallel([
function(callback) { mobike(lat, lng, callback); },
function(callback) { spin(lat, lng, callback); },
],
function(err, results) {
console.log(results);
});
For the parallel method each function only has a callback parameter.
The easiest way to achieve what you want is like this
async.parallel([
function (callback) {
var lng = 0.0
var lat = 0.0
mobike(lat, lng, callback), //This is where you pass in parameters
},
spin
],
function(err, results) {
console.log(results);
});
I tagged Aldo's answer as correct since it was the first response but thanks for your response (would have been just as effective)
– BYates
Jul 3 at 1:24
You can also use bind to pass parameters. Example:
const async = require('async')
const sleep = (ms = 0) => {
return new Promise(resolve => setTimeout(resolve, ms))
}
async.parallel([
mobike.bind(null, 1000), //How or where can I pass these parameters?
spin.bind(null, 500)
],
function(err, results) {
console.log(results);
})
async function mobike (ms) {
await sleep(ms) // pretend to wait for an api request
console.log('mobike waited for ' + ms + ' ms')
return ms
}
async function spin (ms) {
await sleep(ms) // pretend to wait for an api request
console.log('spin waited for ' + ms + ' ms')
return ms
}
Results:
spin waited for 500 ms
mobike waited for 1000 ms
[ 1000, 500 ]
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You can also convert your
async
functions to return apromise
, and usePromise.all
for all output of the functions to achieve the same result.– Brijesh Patel
Jul 3 at 3:35